LearnPython has the Biggest Discount Ever! ------ Get $349 $129 Lifetime Access to all 13 Python Courses
Convert Binary to Decimal Number In Python.
It is a bit straightforward to convert from binary to decimal in Python, but it can also be a little confusing for beginners, especially those who did not understand the conversion formula from the Maths or Computer Science class. In this post, we are going to cover how to do just that, first using the manual method which consists of about 4 steps so that you can understand what happens behind the scenes. After that, we will write python programs to do just the same.
We will first look at how to convert binary to decimal in python without inbuilt function which follows through the steps of manual conversion. But because of differences in the way humans think compared to Python, this process is not so straightforward. For example, in the manual conversion formula, we give our index starting from the right, but Python by default does that starting from the left. So we have to do some kind of reverse engineering.
After that, we then look at how to convert binary to decimal in Python with inbuilt function int()
, which takes in two arguments, the value and the base. In this case, we pass in a binary string and the number 2 (to represent base 2). The int()
function then converts the binary into decimal and returns the integer value.
We will then write a Python program that utilizes the int()
function in order to change binary to decimal. However, we do not use the try and except statements so as to focus on the topic of this post which is to learn how to convert binary to a decimal using Python. If you’re going to write a real-world program that converts binary numbers to decimal numbers, make sure you add these try and except statements. I hope you will learn a lot from this post, leave a comment down below if you have more to ask.
Let’s start by learning what the binary and decimal number systems are.
Table of Contents
- What Is the Binary Number System
- What Is the Decimal Number System
- Steps to convert binary to decimal number manually (Using the Conversion Formula).
- How To Convert From Binary to Decimal in Python Without Inbuilt Function.
- Step 1: Create a variable that holds the binary string.
- Step 2: Convert the binary string into a list.
- Step 3: Reverse the list.
- Step 4: Initialize the variable that holds the sum.
- Step 5: Create a Python for loop that loops over the list, performs exponentiation, and adds to sum.
- Step 6: Print the decimal value to the user.
- Improvements to Python Code to Convert Binary to Decimal Without Inbuilt Function.
- Alternative Method To Convert From Binary to Decimal in Python (Using Inbuilt Function)
- FAQs on Binary to Decimal Conversion in Python
- Conclusion: Binary to Decimal Python.
What Is the Binary Number System
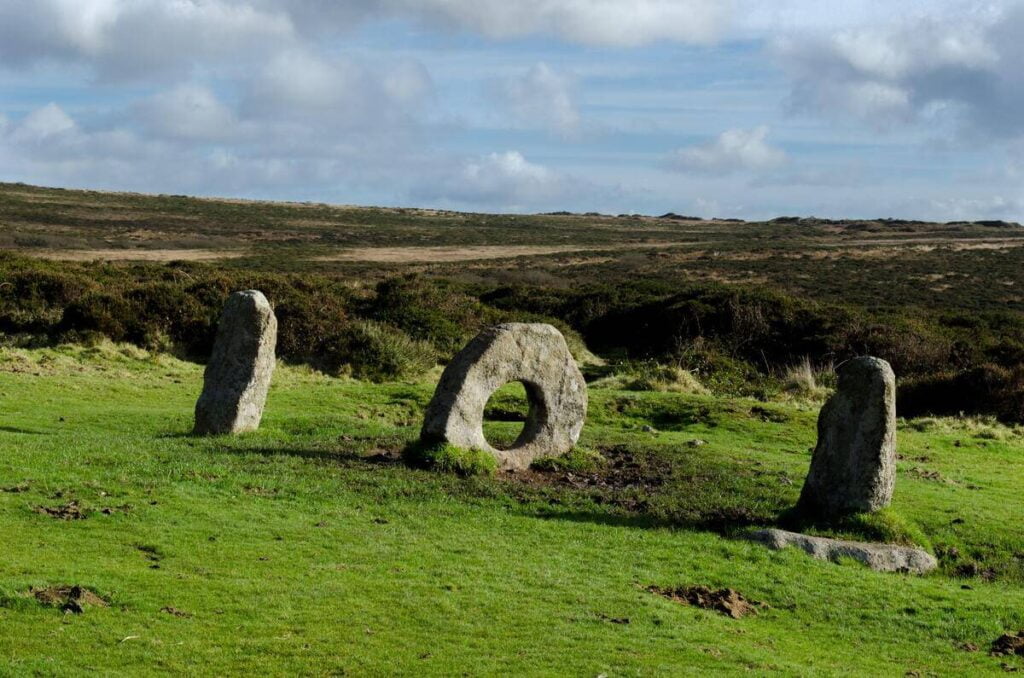
A binary number is a number written in the binary system, also called the “base 2” system. Bi- means two. It uses only two symbols to represent the values of numbers. These two symbols are 1(one) and 0 (zero). In other words, a binary number consists of only 0s and 1s. Binary is the number system that computers can understand. This means that a computer cannot directly interpret any number other than binary digits.
However, humans often want to convert from binary to decimal because decimal is easier to read and use for other mathematical calculations. With Python’s help, it’s easy to convert from binary to decimal.
What Is the Decimal Number System
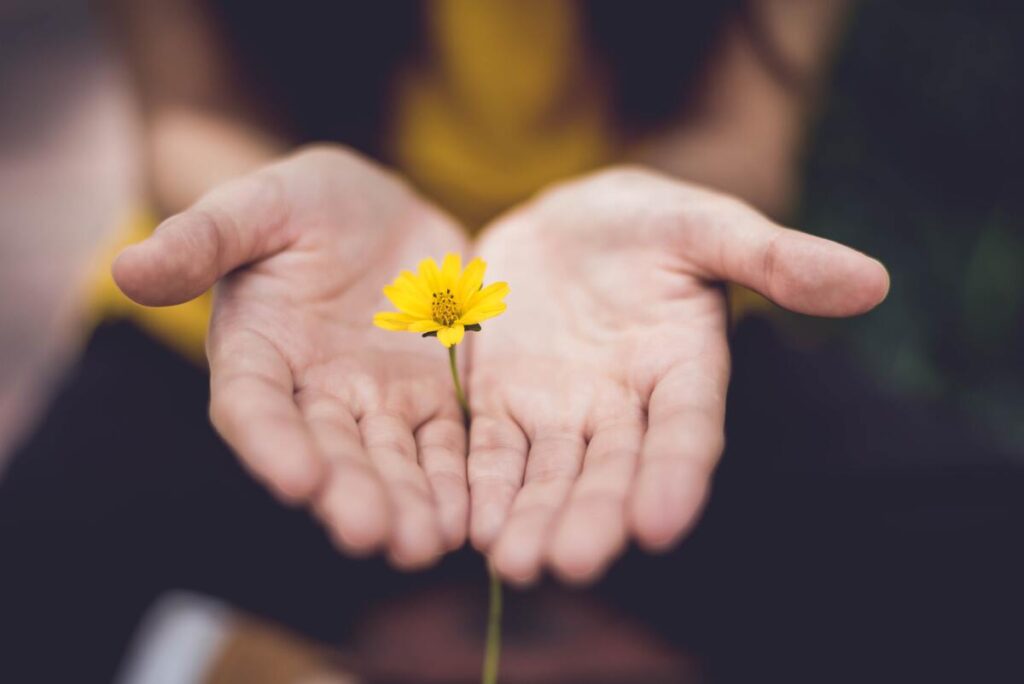
The base 10 number system, also called the decimal number system, is a way to represent numbers with 10 single digits. These numbers are 0, 1, 2, 3, 4, 5, 6, 7, 8, and 9. The decimal number system is probably the one that people use the most. Do not confuse it with decimal numbers, which are numbers with commas, known as floating point numbers in Python. Since the decimal system is more often used, we frequently need to do a binary-to-decimal conversion. Instead of doing this manually, we can write a python program that converts these binary numbers into the decimal number system. We can use it over and over instead of having to do it manually every time we have a binary to be converted into decimal.
But let’s first go over the fundamentals of binary-to-decimal conversion.
Steps to convert binary to decimal number manually (Using the Conversion Formula).
In a Computer Science class, you may be asked to convert a binary number to a decimal using the manual method. If you can make your way to the solution, then you can move to the next section. But if somehow it skipped you, I’ll try my best to make you understand. What I want is for you to understand the underlying theory behind the Python programs we will write to convert binary to decimal. Here we go.
When you have a binary number to convert into a decimal;
- First, give the right-most binary digit an index of zero. Then moving to the left, give each of the next digits the following index, each time incrementing the index by one until you rich the last digit (the left-most binary digit). It may be helpful to write these indexes down, each one below its corresponding digit.
- Next, write down the number 2 raised to the power of each of the indexes below the corresponding indexes. Evaluate the exponentiation.
- After that, multiply each of the results from the previous calculation by the corresponding binary digit.
- And finally, add the values together and you’ll get the value of the decimal number.
For example, let’s say you’re given the binary 1001011 and you’re asked to convert it to decimal manually, here is what you’ll do.
➊ Binary Digits | 1 | 0 | 0 | 1 | 0 | 1 | 1 |
➋ Index of Digit from right to left | 6 | 5 | 4 | 3 | 2 | 1 | 0 |
➌ 2 to the Power Index | 26 | 25 | 24 | 23 | 22 | 21 | 20 |
➍ Exponent Answer multiplied by Binary Digit [row➊ X row➌ ] | (1 × 2⁶) = 64 | 0 | 0 | (1 × 2³) = 8 | 0 | (1 × 2¹) = 2 | (1 × 2⁰) = 1 |
➎ Add these Numbers | 64 + | 0+ | 0+ | 8+ | 0+ | 2+ | 1 |
We have our binary digits in the first row. In the second row ➋, we write the index value of each of our binary digits. Since this is not a calculation, it is not necessary to write it down, I included it to explain where the powers in the third-row ➌ are coming from. Note that we start counting from the right and we count starting at zero. On the third row ➌, we put 2 to the power of each of the indexes. Then on row ➍, we multiply each of our binary digits with its corresponding power of 2. Note that I did not write out multiplication involving the number 0 as they will result in 0. On the fifth row ➎, we add all our results.
If you add all the numbers on the bottom row, you’ll get the answer 75. And that is the decimal equivalent of the binary 1001011. You can use these same steps to convert any binary to decimal values. Now we have to use these same steps in our Python program to convert binary to decimal.
How To Convert From Binary to Decimal in Python Without Inbuilt Function.
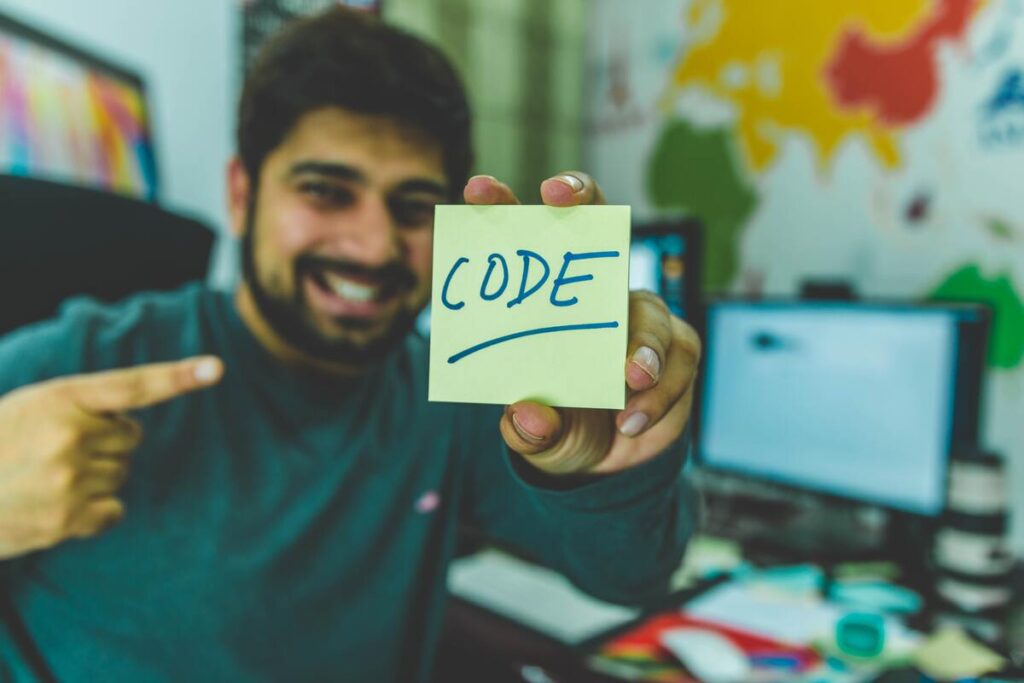
To convert from binary to decimal in Python without Inbuilt function, we create a Python program that follows the steps of the manual conversion. Here is how to do it.
- Create a variable that holds the binary string.
- Convert the binary string into a list.
- Reverse the list since we are counting from the right and going to the left.
- Initialize the variable that holds the sum.
- Create a Python for loop that loops over the list, each time performing the exponentiation and adding to the value in variable sum.
- Print the decimal value to the user.
Below is how to write a python program to convert binary to decimal. The program below is a typical program to convert binary to decimal in python without inbuilt function. It follows the steps of manual conversion that we looked at in the introduction. I encourage you to take a look at it in order to understand what is going on behind the scenes of other built-in functions or the best program to convert binary into decimal:
➊binary_str = input('Enter a Binary number to convert it to Decimal: ')
➋digit_list = list(binary_str)
➌digit_list.reverse()
➍sum = 0
for digit in ➎(range(len(digit_list))):
➑sum = sum + ➐int(digit_list[digit]) * ➏pow(2, digit)
➒print(sum)
> Enter a Binary number to convert it to Decimal: 1001011
75
Let’s explain what is happening in detail.
Step 1: Create a variable that holds the binary string.
First, we get the binary number from the input. We do that using the input()
function. We store the string in a variable called binary_str
.
Step 2: Convert the binary string into a list.
In order to be able to iterate through each of the digits of the binary, we have to convert it into a list. We do that at ➋ by using the list()
function.
Step 3: Reverse the list.
From the case study in the introduction, we learned that we have to assign our binary indexes starting from the right. This is extremely difficult to do using a for loop in Python. Because Python starts reading from the left, a much simpler way is to reverse the list itself. That is the reason you see the reverse()
function at three. The good thing is Python also reads list indexes starting from zero, so there is nothing more we have to do.
Step 4: Initialize the variable that holds the sum.
At ➍, we initialize the variable that will hold the total sum of the products of a binary digit and the power of 2 to the exponent of the index.
Step 5: Create a Python for loop that loops over the list, performs exponentiation, and adds to sum.
We then start our for-loop. Let’s pay close attention to ➎. We use the len()
function to get the number of items in the digit_list
list. We use the range()
function to get all numbers between the first index (0) and the last. Each of these numbers is the index of a corresponding digit. And through each iteration of the list, it will be stored in the digit variable.
At ➑, we append the sum of the products of a binary digit and the power of 2 to the exponent of the index to the sum variable. Let’s use one of the indexes as an example. So for the first digit in the reversed list, we have '1'
. Its index is 0
. The expression digit_list[digit]
, in this case, is like digit_list[0]
which will gives us '1'
.
Since this is a string, we have to change it into an integer at ➐ in order to be able to perform accurate math operations with it. That is why we use the int()
function. We now have 1
, and we want to multiply it by 2 to the exponent of its index. At ➏, we perform the multiplication using the multiplication operator (*
) and the exponentiation using the pow()
function which takes in the base as its first argument and the exponent as its second. We can also use the exponentiation operator (**
) to perform exponentiation.
Step 6: Print the decimal value to the user.
At ➒, we use the print()
function outside the for-loop to print the total sum of additions to the sum variable that we get after every digit in the list gets the exponentiation operation.
Improvements to Python Code to Convert Binary to Decimal Without Inbuilt Function.
Here are some improvements you can make to the code we documented above.
- Use try and except, if statements, or any other way to check if the input given by the user is a valid binary number.
- Use the trimming functions to trim the input given by the user in case it contains some whitespace.
Alternative Method To Convert From Binary to Decimal in Python (Using Inbuilt Function)
In this section, we will look at how to convert binary to decimal in Python with inbuilt function. This inbuilt function is the int()
function, which takes in two arguments, first the value to be converted and second the base to which the value belongs. In this case, we will pass in the binary string and the number 2 to represent base 2. The int()
function then converts the binary into decimal and returns the decimal value.
In the previous code, we wrote custom Python code to convert from binary to decimal. It turns out, Python has a built-in function that can do the work for us automatically. This function is int()
. Most of us know that the int()
function is used to convert a non-integer datatype into an integer, be it a string, a floating point number, or a complex number. We can also use this function to change binary to decimal.
As an example, I will pass in '1001011'
as my first argument into the int()
function to convert it from its binary form into a decimal number. Then I will pass in 2 as my second because this is the base where my first argument belongs. Let’s take a look at an example below:
>>> int('1001011', 2)
75
Note that the first argument should be a string. If you use any other datatype other than that you’ll get the int() can't convert non-string with explicit base
error. This is the fastest, easiest, and most pythonic way to convert binary to decimal in Python.
Above, we tested the int()
function in the Python Interactive Shell, Now let’s use the function to write a Python program to convert binary to decimal. The program below is a typical program to convert binary to decimal in Python with an inbuilt function. It uses a function that’s already in the Python programming language.
binary_str = input("Enter a Binary number to convert it to Decimal :")
decimal_num = int(binary_str, 2)
print(decimal_num)
Here is the output of the code above. We convert 1001011 into its decimal equivalent.
>>> Enter a Binary number to convert it to Decimal : 1001011
75
If you are going to use this program in real-world projects, make sure you add in try and except statements so that the program can be sure if the user has inputted binary numbers, instead of other numbers that are not binary.
FAQs on Binary to Decimal Conversion in Python
What is bin() function in Python?
The bin()
function, short for binary, is a Python function that does the opposite of what we were doing in this post, that is, Instead of converting binary to decimal, it takes in a decimal number as its argument, and converts it from decimal to binary.
How do you convert binary equivalent 10101 to a decimal? How do you turn 1011 into a decimal?
Follow the steps in this guide to convert from binary to decimal, you can either do it manually, or you can write a Python program to convert binary to decimal. You should get the decimal equivalent of 10101 to be 21 and that of 1011 to be 11.
Conclusion: Binary to Decimal Python.
Congrats on going through this post. In this post, we covered how to convert binary to decimal, first in a manual way so that you can understand the behind-the-scenes. We then wrote Python programs to do just the same. We first looked at how to convert binary to decimal in Python without an inbuilt function which follows through the steps of manual conversion. We then looked at how to convert binary to decimal in Python with an inbuilt function.
We then wrote a Python program that utilized the int() function in order to change binary to decimal. However, we did not use the try and except statements to focus on the topic of this post which is to learn how to convert binary to a decimal using Python. If you’re going to write a real-world program that converts binary numbers to decimal numbers, make sure you add these try and except statements.
Otherwise, I hope you learned a lot from this post, leave a comment down below if you have more to ask. Other than that, thank you for stopping by, I’ll meet you in other CodingGear articles. Peace!