LearnPython has the Biggest Discount Ever! ------ Get $349 $129 Lifetime Access to all 13 Python Courses
How to Find the Roots of a Quadratic Equation in Python
A quadratic equation is a mathematical function that takes the form ax2 + bx + c = 0, where a, b, and c are constants. The roots of a quadratic equation in simple terms are the numbers for which x is standing for. There should always be 2 roots for a quadratic equation (sometimes those roots can be of the same value). In mathematics, we can find the roots of a quadratic equation using the Quadratic formula:
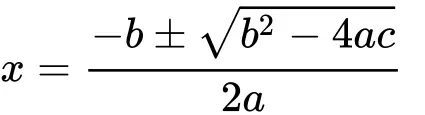
We can create a program to find the roots of a Quadratic equation in Python by writing Python code that imitates the Quadratic formula.
This post will show you how to do that.
Find Roots of Quadratic Equation Python: Table of Contents
- How to Find the Roots of a Quadratic Equation in Python
- Python Program To Find The Roots Of Quadratic Equation
How to Find the Roots of a Quadratic Equation in Python
I am going to break this into steps. Then in the next section, I’ll show you the full Python code.
When given the following quadratic equation of the form ax2 + bx + c = 0 and you’re asked to write a Python program to find the roots(solution) of the equation, the best way to go about it is to follow the steps below:
- Store the values of a, b, and c in variables
- Create a new variable that stores the square root of the discriminant
- Write the math expressions to solve the roots (x1, x2)
- Display the Output
Step 1: Store the values of a, b, and c in variables

Depending on where we will be getting the quadratic equation from, we have to store each constant in a variable. We can either write code that asks the user to type in the values of the constants or the values can just be coming from within the Python program.
a = float(input("The value of a: "))
b = float(input("The value of b: "))
c = float(input("The value of c: "))
Since the value that we get from the input()
is always a string, we have to change it into a floating point number so that we can perform mathematical operations on them. We do this in Python using the float()
function.
Step 2: Create a new variable that stores the square root of the discriminant
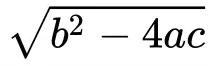
Since both equations contain the part shown above, known as the square root of the discriminant, we can store that value in a variable to reduce the amount of code we will have to write. Let’s store it in a variable called sqr_discriminant
.
sqr_discriminant = (➊b**2 - ➋4*a*c)➌**0.5
At ➊, we use the exponentiation operator (**
) to raise b to the power of 2. In other words, we are squaring the value of b. We can as well use the pow()
function which takes in the base and the power such that using it should have made that part of our code look like this: pow(b, 2)
At ➋, we multiply 4 by a by c using the multiplication operator.
Last by not least, at ➌, we once again use the exponentiation operator on our discriminant to raise it to the power of 0.5
or ½. This is exactly similar to finding the square root. If you do not know about this, watch the video below.
Look back at our code: Note that we use the brackets so that the expression in the brackets can be calculated first. Then after that, we find the square root of the result. This follows the PEMDAS rule.
Step 3: Write the math expressions to solve the roots (x1, x2)

The square of the discriminant has been taken care of, what’s left is to write the full expressions to solve the values of x1 and x2. The Quadratic formula can be divided into two formulas since there should be two roots. One is adding to the square of the discriminant, and the other is subtracting. In Python, we write this as follows:
x1 = (-b + sqr_discriminant)/(2*a)
x2 = (-b - sqr_discriminant)/(2*a)
We use the division operator to divide the above expression with 2a.
The rest of the math operators I’m pretty sure are quite understandable.
Step 4: Display the Output
At this point, the quadratic equation has been already solved by the few Python lines of code we have written so far. What’s left now is to display the results back to the user or console. We use the print()
function to do that in Python.
print(f'x1 = {x1}')
print(f'x2 = {x2}')
We use Python f strings to format the output.
Python Program To Find The Roots Of Quadratic Equation
The Python code below is what we will get after combining the steps on how to find all roots of a quadratic equation in Python. We combine all the steps in a function called roots()
, which takes in three arguments a, b and c. Then it returns the two roots for that quadratic equation.
def roots(a, b, c):
sqr_discriminant = (b**2 - 4*a*c)**0.5
x1 = (-b + sqr_discriminant)/(2*a)
x2 = (-b - sqr_discriminant)/(2*a)
print(f'x1: {x1}')
print(f'x2: {x_2}')
a = float(input("The value of a: "))
b = float(input("The value of b: "))
c = float(input("The value of c: "))
roots(a, b, c)
First, we define the function roots()
using the keyword def. The function takes in the three constants of a quadratic formula and returns the roots of that quadratic equation.
Next, we get the values of the three constants from the user and convert them into floating-point numbers.
Then, we pass these three constants to the roots()
function.
With the given values of a, b, and c, this function solves for the roots of the quadratic formula and outputs them.
The program, when run, prompts the user to enter the values of a, b, and c that correspond to the quadratic equation whose roots they want to solve for.
Let’s try to solve the quadratic equation x2 + 5x + 6 = 0
using the Python program we have just created.
> The value of a: 1
> The value of b: 5
> The value of c: 6
x1 = -2.0
x2 = -3.0
And with that, we wrap up our tutorial on how to find the roots of a quadratic equation in Python. I hope you learned a lot. If you have any questions, feel free to use the comments section below. Peace!