LearnPython has the Biggest Discount Ever! ------ Get $349 $129 Lifetime Access to all 13 Python Courses
How to Check If a List Is Empty in Python
Have you ever encountered a situation where you need to perform some operations on a list, but only if it has some elements in it?
For example, imagine you are developing a shopping cart app, and you want to calculate the total price of the items in the cart. However, if the cart is empty, you don’t want to do any calculations, but instead display a message to the user that the cart is empty. How can you check if a list is empty in Python?
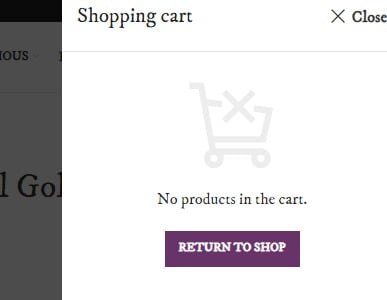
In this blog post, we will explore different ways of checking if a list is empty in Python, and discuss their advantages and disadvantages. We will cover the following methods:
Methods for Checking Empty Lists:
Method 1: Using the not Operator (Most Pythonic):
The most Pythonic and recommended way of checking if a list is empty is to use the not
operator. This operator returns True
if the operand is False
, and False
if the operand is True
. In Python, an empty list evaluates to False
, while a non-empty list evaluates to True
. Therefore, we can use the not
operator to check if a list is empty or not.
For example, suppose we have a list called my_list
, and we want to print its elements if it is not empty, or print “Empty list” otherwise. We can use the following code:
my_list = [1, 2, 3] # change this to [] to test an empty list
if not my_list:
print("Empty list")
else:
print(my_list)
The output of this code is:
[1, 2, 3]
If we change my_list
to []
, the output becomes:
Empty list
This method is simple and readable, and it follows the principle of “truthiness” in Python, which means that objects that are considered empty or zero are treated as False
, while objects that are considered non-empty or non-zero are treated as True
.
Method 2: Using the len() Function:
Another way of checking if a list is empty is to use the len()
function. This function returns the number of elements in a list (or any other iterable object). Therefore, we can check if a list is empty by comparing its length to zero.
For example, using the same my_list
as before, we can use the following code:
my_list = [1, 2, 3] # change this to [] to test an empty list
if len(my_list) == 0:
print("Empty list")
else:
print(my_list)
The output of this code is the same as before:
[1, 2, 3]
If we change my_list
to []
, the output becomes:
Empty list
The advantage of using the len()
function is that it is clear and explicit. It also works with any data structure that supports the len()
function, such as tuples, sets, dictionaries, strings, etc. Therefore, it is more versatile than using the not
operator.
Method 3: Direct Comparison to an Empty List:
A third way of checking if a list is empty is to compare it directly to an empty list using the ==
operator. This operator returns True
if both operands are equal, and False otherwise. Therefore, we can check if a list is empty by comparing it to []
.
For example, using the same my_list
as before, we can use the following code:
my_list = [1, 2, 3] # change this to [] to test an empty list
if my_list == []:
print("Empty list")
else:
print(my_list)
The output of this code is again the same as before:
[1, 2, 3]
If we change my_list
to []
, the output becomes:
Empty list
The advantage of using direct comparison to an empty list is that it is very clear and intuitive. It also works with any data structure that supports the ==
operator, such as tuples, sets, dictionaries, strings, etc. Therefore, it is also very flexible.
However, there is a potential drawback of using direct comparison to an empty list: performance. According to some benchmarks, using direct comparison to an empty list can be slower than using the not
operator or the len()
function in some cases. This is because direct comparison involves creating an empty list object and comparing each element of the original list with it. This can be costly if the original list is large or contains complex objects.
Therefore, direct comparison to an empty list should be avoided in performance-critical situations, and instead, use the not
operator or the len()
function.
When to Check for Empty Lists:
Checking for empty lists is a common task in Python programming, as it can help us prevent errors and implement conditional logic based on the contents of a list. Some common scenarios where checking for empty lists is essential are:
Preventing errors when iterating over or accessing elements:
If we try to loop over an empty list using a for
loop or access an element using indexing or slicing, we will get an error. For example:
my_list = []
for item in my_list:
print(item) # raises an error
print(my_list[0]) # raises an error
To avoid these errors, we can check if the list is empty before iterating over it or accessing its elements.
Implementing conditional logic based on list contents:
Sometimes we want to perform different actions depending on whether a list has any elements or not. For example, we may want to display a message if the list is empty, or process the elements if the list is not empty. For example:
my_list = ["apple", "banana", "orange"]
if not my_list:
print("The list is empty")
else:
print("The list has", len(my_list), "elements")
Optimizing code by avoiding unnecessary operations on empty lists:
Sometimes we may have some operations that are costly or time-consuming, and we want to avoid them if the list is empty. For example, we may want to sort a list, but sorting an empty list is pointless and wasteful. Therefore, we can check if the list is empty before sorting it. For example:
my_list = []
if not my_list:
print("The list is empty")
else:
my_list.sort()
print("The sorted list is", my_list)
Best Practices:
As we have seen, there are different ways to check if a list is empty in Python, and each one has its own advantages and disadvantages. However, some methods are more preferred and recommended than others, based on their readability, performance, and idiomaticity. Here are some best practices for checking empty lists in Python:
- Use the
not
operator as the most Pythonic and readable approach for most cases. This method is simple, elegant, and follows the principle of truthiness in Python. - Use the
len()
function when clarity or flexibility with other data structures is required. This method is explicit and versatile, and it can be used for checking the lengths of other iterables besides lists. - Avoid direct comparison to an empty list for performance-critical situations. This method is clear but potentially slower than the other methods, especially for large lists. It is also considered less idiomatic and less readable than using the not operator.
Conclusion:
In this blog post, we have learned how to check if a list is empty in Python using different methods: the not
operator, the len()
function, and direct comparison to an empty list. We have also discussed when and why we need to check for empty lists, and what are the best practices for doing so.
Checking for empty lists is a common and important task in Python programming. By following these techniques and tips, you can write more robust and efficient code that handles empty lists gracefully.
We hope you enjoyed this blog post and learned something new.
Happy coding!