LearnPython has the Biggest Discount Ever! ------ Get $349 $129 Lifetime Access to all 13 Python Courses
How to Setup Environment Variables in Django
In a Django project, some information needs to be kept secret; like a Secret key, a Database username and password, and API keys. This is because their exposure to foreign parties can put your project at risk of security attacks, especially if you upload your Django project to Git Hub or somewhere similar. In Django projects that are not meant for deployment, this may not be a big issue but for production-level projects, it is mandatory to keep the information safe. In Django, we do that by using environment variables.
Environment variables are variables stored in the system of a computer so that no one can access them. This post will elaborate more on how to set up environment variables in your Django project.
How to Set Environment Variables in Django
Follow these steps to use Environment variables in a Django project:
Step 1: Install python-dotenv
The tool we are going to use to set our environment variables in this post is python-dotenv.
Python-dotenv can function by reading key-value pairs from a .env
file, and it can then use those pairs to set environment variables.
To install it in your Django project, run the following command in the terminal of your Django project:
(env) $ pip install python-dotenv
Once the command has been run, it’s now time to use it in the settings.py
file of our Django project.
Step 2: Import and Initialize python-dotenv
in settings.py
We start by importing python-dotenv
in our settings.py
file. At the top of settings.py
file below the Path import, add the following 2 import statements and an initialization statement:
from dotenv import load_dotenv
import os
load_dotenv()
load_dotenv
is going to load our environment variables from the .env
file. os
is going to access the operating system since we’re saving these variables to the system on the computer.
Below the imports, we initialize dotenv by calling the load_dotenv()
function.
We can now start using python_dotenv in the file.
Step 3: Create a .env
file at the root of the Project
At the root of your Django project folder, create a new file called .env
. Make sure you don’t miss the period at the beginning. Your project structure should look like this:
...
├── .env #here
└── manage.py
This is a special type of file. Your code editor should display it with an icon that is different from the rest. In VS Code, it is displayed as a gear icon.
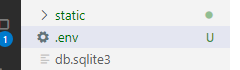
Step 4: Set Environment Variables in .env
file
Now it is time to declare all the variables that you want their values to be kept safe or secret. Such information can be passwords, secret keys, API keys, and so on. As an example, I will list a few variables that are important to keep safe. The list can be longer depending on your project:
SECRET_KEY=django-insecure-g6owp@47mbu33+nemhf$btj&6e7t&8)&n!uax1obkf-d)9$9*j
DB_NAME=moviereviews
DB_USER=root
DB_PASS=hO5xY%00j
These are a few of the variables you can add to the .env
file.
Note that we don’t use quotes around strings because they will be converted automatically when they get loaded into the settings.py file.
Note that we also do not use spaces on both sides of the assignment operator because there is no need to.
You can learn more about how to declare environment variables using python_dotenv from its pypi page.
Step 5: Assign the Environment Variables in the settings.py
Now that our variables are in the .env file, it’s time to replace the explicit values in the settings.py
with the ones in the Django environment variables file.
We use environ from os as follows:
SECRET_KEY = os.environ.get('SECRET_KEY') #here
...
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': os.environ.get('DB_NAME'), #here
'USER': os.environ.get('DB_USER'), #here
'PASSWORD': os.environ.get('DB_PASS'), #here
'HOST': '127.0.0.1',
'PORT': '3306',
'OPTIONS': {'init_command': "SET sql_mode='STRICT_TRANS_TABLES'"},
}
}
We use the get()
method to get the environment variable from the .env
file.
The DATABASES
dictionary in this example has the configuration for the MySQL database.
To check if you’ve set the environment variables correctly, run the development server of your Django project.
Step 6: Add the .env
file to .gitignore
file
Since the .env
file contains sensitive information about our Django project, it should also not be uploaded to a git repository. To do that, you have to add it to the list in the .gitignore
file of your Django project. .gitignore
is a file where you list all the files and directories you do not want to be uploaded to git.
Conclusion
I hope you learned good information about Django environment variables, if you have any questions or suggestions, let me know in the comments section.