LearnPython has the Biggest Discount Ever! ------ Get $349 $129 Lifetime Access to all 13 Python Courses
What Is Casting In Python
Type casting, or just “casting,” in Python refers to the process of converting one data type to another. For example, converting an integer to a string, a float to an integer, or a string to a list, etc.
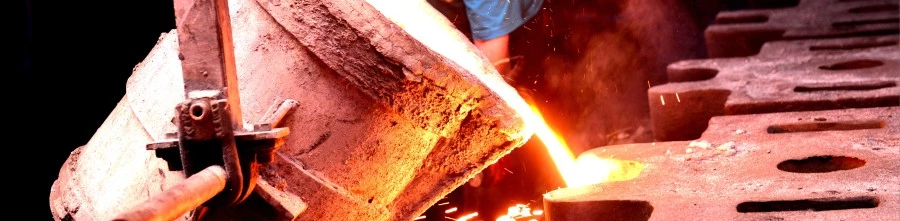
The word “casting” in programming comes from the metalworking process of “casting,” in which molten metal is poured into a mold to make a certain shape. Similarly, when you cast a value in Python from one data type to another, you’re transforming the value into a new shape, or in this case, type, to meet your needs.
In addition to “casting,” other terms that are used to describe the process of converting one data type to another in programming include type conversion, type coercion, or type transformation.
How To Do A Type Cast In Python
To do a type cast, Python offers some built-in functions which you can use to convert your desired datatype into another. The most common of those functions are displayed in the table below:
From the list above, you can see that the name of the function is derived from the type a value is going to convert into.
Follow the links to go to an in-depth tutorial for each of the functions concerned or to learn alternatives to each method. Alternatively, you can also use the example I’m going to illustrate below to get a general idea of how the functions are used.
Python Example of Type Casting (string to int)
For this example, I’m going to demonstrate how to convert a string (of type str()
) into an integer (of type int()
). The illustration applies to the rest of the functions.
x = "10"
y = int(x)
print(y) # Output: 10
In the code above, x
stores the value'10'
. Although 10
itself is a number, the type of x
is str()
because the 10
is wrapped in quotes. On line 2, we use the int() function to convert the value of x into an integer, and we store the cast value in the y variable. Finally, we print the value to the console.
FAQ: what happens if you cast a blank variable in python?
If you try to cast a blank or None
value in Python, you will get a TypeError telling you that a value to be cast must not be of type None. For example, if you try to cast a variable with a None value you’ll get the TypeError: int() argument must be a string, a bytes-like object or a real number, not 'NoneType'
. This will also happen for other casting functions as well.
Thats it for this post, I’ll see you in others.