LearnPython has the Biggest Discount Ever! ------ Get $349 $129 Lifetime Access to all 13 Python Courses
Reset Django Admin/Superuser Password
If you have forgotten the password for your Django project’s Admin or Superuser, you do not have to create another superuser. As long as you remember the Superuser’s username or you are still logged in to the admin panel, then you can easily reset the Django admin’s password using the methods that I’ll share in this post.
Table of Contents: Reset Django Admin/Superuser Password
- Reset Django Superuser Password Using the changepassword command
- Using the set_password() function in Shell or Code.
- Reset Django Superuser Password Using the Django admin
Reset Django Superuser Password Using the changepassword
command
The fastest method to reset your Django admin or superuser password is to use the python manage.py changepassword
command. All you have to do is to run the command in your Django project terminal and Django will prompt you to enter a new password. After that, Django will print out ‘Password changed successfully for user 'username'
‘ to show that the password for the user has been reset successfully.
Requirements
- Know Your Superuser Username, Not Required if you have one Superuser
Here are the steps to reset the admin password of your Django project using changepassword
command:
Step 1: Open the terminal and run python manage.py changepassword
Open the terminal and cd
(change directory) into the project folder (the directory with the manage.py
file). Then run the command python manage.py changepassword (username)
. Make sure the virtual environment is activated before you run the command.
$ python manage.py changepassword (username)
Changing password for user 'username'
Password:
Password (again):
Password changed successfully for user 'username'
In this case, (username)
is the username for your Django project’s superuser. Note that the password won’t appear in the console as you type it. This is done for security reasons.
If your project has only one superuser, then that’s a good thing because you no longer have to specify the username when you run the command. It’s a good thing because you may also have forgotten both the password and the username, so running it without specifying the username will work just as fine.
$ python manage.py changepassword
However, if you run this command without specifying the username when there are many superusers in your Django project, then Django will apply the operation for the currently logged-in superuser.
Step 2: Type in and Confirm the new password.
Changing password for user 'username'
Password:
Password (again):
Password changed successfully for user 'username'
This time, type in a password that you will remember. As you are typing this password, Django will not print any characters on the terminal for security reasons. Next, you will have to type in the same password again to confirm that you know it.
Django will then print out the message ‘Password changed successfully for user 'username'
‘ to show that the superuser’s password has been reset.
Using the set_password()
function in Shell or Code.
You can use the set_password()
function in the shell or in Python code to reset the superuser’s password. Here’s how to do it:
Requirements
- Know Your Django Superuser Username
Step 1: Open the Python Interactive Shell
You can do this in Django by running the following command in your Django project’s terminal:
$ python manage.py shell
To indicate that you’re now in the shell, there will be >>>
at the start of each line in the console.
Step 2: Select the superuser you want to reset the password for
We are going to access the list of all created superusers using the following code:
>>> from django.contrib.auth import get_user_model
>>>list(get_user_model().objects.filter(is_superuser=True).values_list('username', flat=True))
At 1, we import the get_user_model
so that we can access the list of users that we have. Now what we have to do is to filter the users who are of type superuser. We do that by setting is_superuser = True in our filter. We further narrow down our filter by typing in the username of the superuser whom we want to reset the password for. What is left now is to change the password for that admin using the set_password function.
user = get_user_model().objects.get(username=u)
We select our user, and we store him in the user variable.
Step 3: Change the password using set_password()
user.set_password(password)
Then we use the set_password
function on our user object and we pass in the new password that we want. Now what’s left is to save.
Step 4: Save the newly created password
user.save()
If you are going to use this functionality in your code instead of the Interactive shell, you can write a function that utilizes all we have done above to do just the same:
Here is an example:
from django.contrib.auth import get_user_model
def reset_password(u, password):
try:
user = get_user_model().objects.get(username=u)
except:
return "User could not be found"
user.set_password(password)
user.save()
return "Password has been changed successfully"
We have created a function called, which takes in a username and a password. If the user exists, the password of that user will be reset by the one that was passed in the function and it will be saved into the database. If the user does not exist, the function will return an error.
Reset Django Superuser Password Using the Django admin
The last method to reset a Django superuser’s password is to use the admin panel. This method only works if the user is already logged into the admin panel. Note that the superuser who wants his password to be reset is the one who should be logged in. This is because other users do not have permission to change other users’ passwords.
This method requires you to know the old password of your superuser.
Requirements:
- Know your old superuser password
- Should be logged in to the admin panel or Know your login details
Here are the steps to take:
Step 1: Run the server and Navigate to http://127.0.0.1:8000/admin
Before you navigate to the localhost, you have to make sure your Django project server is running. If you are already logged in, you should see the dashboard immediately, but if you’re not, you’ll be redirected to http://127.0.0.1:8000/admin/login/?next=/admin/
and you’ll be prompted to enter the login details. In that case, you can no longer use this method to reset your admin password.
Step 2: Click on CHANGE PASSWORD
At the top right corner of the Django admin, click the CHANGE PASSWORD link.

You will be taken to a form where you type in your old password and new password.

Step 3: Type In your Old Password
Unlike other methods for resetting your superuser’s password, this one requires you to know the old password for your user. This is done for security reasons. If you have forgotten the old password, unfortunately, you cannot continue with this method.
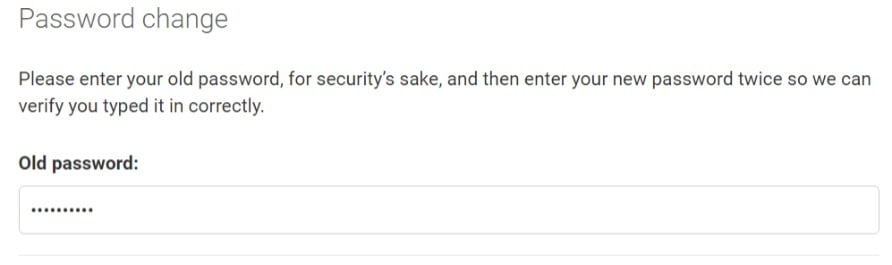
Step 4: Type in and Confirm the new password.
Next, type in your password. There are two forms where you have to enter in the same password, the first one for the new password, and the second for the confirmation of the new password.
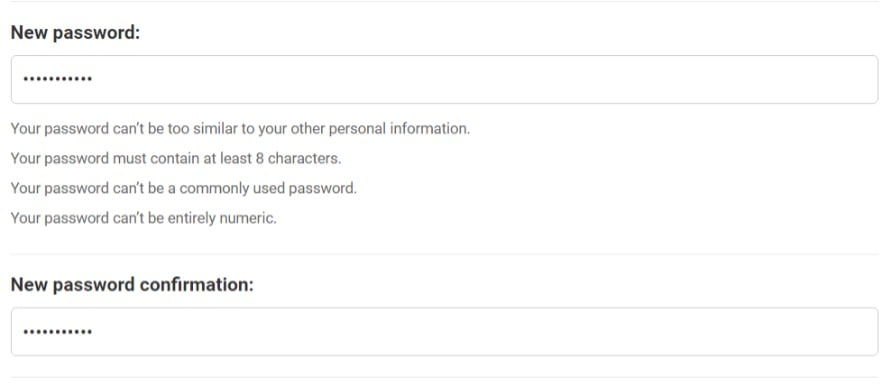
Read the guidelines below the New password form to learn how you should construct your new password.
Step 5: Click the CHANGE PASSWORD button
Last but not least, click the CHANGE PASSWORD button at the bottom right corner of the form.

If your password has been reset successfully, you’ll be redirected to a page that says ‘Password change successful’:
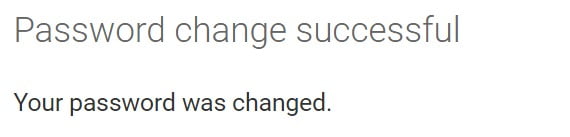
And those my friends, are the ways to reset your Django superuser password.